I have changed the design of the Adaptive Card and adapted the link for IcingaDB.
With the imageurl variable you can display an image with the corresponding color (Important: The image must be accessible from the Internet for teams).
The author and comment are only displayed if you have really commented something.
With “msteams”: {“width”: “Full”} the maximum width in teams is used.
Hope this helps someone
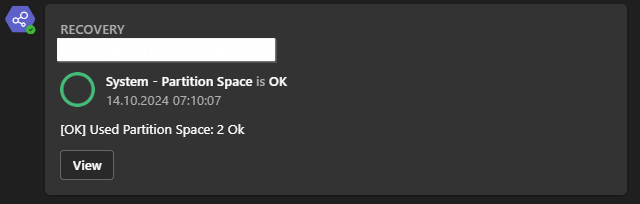
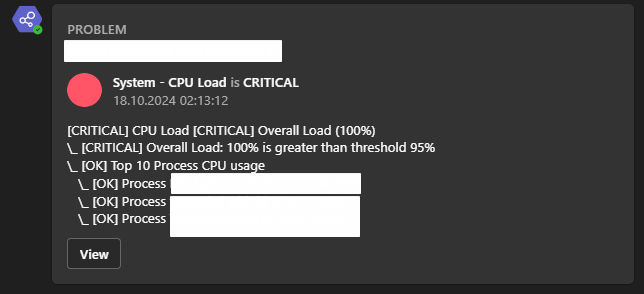
Service
#!/usr/bin/env python3
import requests, os, sys
import urllib3, argparse
from datetime import datetime
urllib3.disable_warnings()
current_datetime = datetime.now()
current_datetime_str = current_datetime.strftime("%d.%m.%Y %H:%M:%S")
parser = argparse.ArgumentParser()
parser.add_argument('-4', help='hostaddress4')
parser.add_argument('-6', help='hostaddress6')
parser.add_argument('-H', help='hostaddress')
parser.add_argument('-b', help='author')
parser.add_argument('-c', help='comment')
parser.add_argument('-d', help='datetime')
parser.add_argument('-e', help='servicename')
parser.add_argument('-l', help='hostname')
parser.add_argument('-n', help='hostdisplayname')
parser.add_argument('-o', help='output')
parser.add_argument('-p', help='pager')
parser.add_argument('-s', help='state')
parser.add_argument('-t', help='type')
parser.add_argument('-u', help='servicedisplayname')
parser.add_argument('-v', help='logtosyslog')
parser.add_argument('-i', help='icingaweburl')
parser.add_argument('-g', help='grafanaurl')
args = parser.parse_args()
hostaddress = args.H
author = args.b
hostname = args.l
comment = args.c
hostdisplayname = args.n
state = args.s
output = args.o
servicename = args.e
boturl = args.p
servicedisplayname = args.u
notificationtype = args.t
icingaweburl = args.i
grafanaweburl = args.g
if state == 'OK':
color = 'good'
imageurl = "https://i.imgur.com/yLPKBlF.png"
elif state == 'UP':
color = 'good'
imageurl = "https://i.imgur.com/yLPKBlF.png"
elif state == 'WARNING':
color = 'warning'
imageurl = "https://i.imgur.com/GIv0R4o.png"
elif state == 'DOWN':
color = 'attention'
imageurl = "https://i.imgur.com/C2B8moA.png"
elif state == 'CRITICAL':
color = 'attention'
imageurl = "https://i.imgur.com/C2B8moA.png"
elif state == 'UNKNOWN':
color = 'light'
imageurl = "https://i.imgur.com/ALr2Cwx.png"
elif state == 'UNREACHABLE':
color = 'light'
imageurl = "https://i.imgur.com/ALr2Cwx.png"
else:
color = 'dark'
imageurl = "https://i.imgur.com/ALr2Cwx.png"
# Check if type is custom
if comment or author:
iscomment = "true"
else:
iscomment = "false"
headers = {
'Content-Type': 'application/json;charset=utf-8',
}
json_data = {
"type":"message",
"attachments":[
{
"contentType":"application/vnd.microsoft.card.adaptive",
"contentUrl":"null",
"content":{
"$schema":"http://adaptivecards.io/schemas/adaptive-card.json",
"type":"AdaptiveCard",
"version":"1.3",
"title":"Monitoring",
"msteams": {
"width": "Full"
},
"body":[
{
"type": "Container",
"items": [
{
"type": "TextBlock",
"text": notificationtype,
"weight": "bolder",
"size": "medium",
"isSubtle": "true",
"spacing": "None"
},
{
"type": "TextBlock",
"text": hostdisplayname,
"weight": "bolder",
"size": "large",
"spacing": "None"
},
{
"type": "ColumnSet",
"columns": [
{
"type": "Column",
"width": "auto",
"items": [
{
"type": "Image",
"url": imageurl,
"altText": state,
"size": "small",
"style": "person"
}
]
},
{
"type": "Column",
"width": "stretch",
"items": [
{
"type": "RichTextBlock",
"inlines": [
{
"type": "TextRun",
"text": servicedisplayname,
"weight": "bolder",
"wrap": "true"
},
{
"type": "TextRun",
"text": " is ",
"weight": "bolder",
"wrap": "true",
"isSubtle": "true"
},
{
"type": "TextRun",
"text": state,
"weight": "bolder",
"wrap": "true"
}
]
},
{
"type": "TextBlock",
"spacing": "none",
"text": current_datetime_str,
"isSubtle": "true",
"wrap": "true"
}
]
}
]
}
]
},
{
"type": "Container",
"items": [
{
"type": "RichTextBlock",
"inlines": [
{
"type": "TextRun",
"text": output
}
]
},
{
"type": "FactSet",
"isVisible": iscomment,
"facts": [
{
"title": "Comment:",
"value": comment
},
{
"title": "Author:",
"value": author
}
]
}
]
}
],
"actions": [
{
"type": "Action.OpenUrl",
"url": icingaweburl+"/icingadb/service?name="+servicename+"&host.name="+hostname+"",
"title": "View"
}
]
}
}
]
}
response = requests.post(
boturl,
headers=headers,
json=json_data,
verify=False
)
Host
#!/usr/bin/env python3
import requests, os, sys
import urllib3, argparse
from datetime import datetime
urllib3.disable_warnings()
current_datetime = datetime.now()
current_datetime_str = current_datetime.strftime("%d.%m.%Y %H:%M:%S")
parser = argparse.ArgumentParser()
parser.add_argument('-4', help='hostaddress4')
parser.add_argument('-6', help='hostaddress6')
parser.add_argument('-H', help='hostaddress')
parser.add_argument('-b', help='author')
parser.add_argument('-c', help='comment')
parser.add_argument('-d', help='datetime')
parser.add_argument('-l', help='hostname')
parser.add_argument('-n', help='hostdisplayname')
parser.add_argument('-o', help='output')
parser.add_argument('-p', help='pager')
parser.add_argument('-s', help='state')
parser.add_argument('-t', help='type')
parser.add_argument('-v', help='logtosyslog')
parser.add_argument('-i', help='icingaweburl')
parser.add_argument('-g', help='grafanaurl')
args = parser.parse_args()
hostaddress = args.H
author = args.b
hostname = args.l
comment = args.c
hostdisplayname = args.n
state = args.s
output = args.o
boturl = args.p
notificationtype = args.t
icingaweburl = args.i
grafanaweburl = args.g
if state == 'OK':
color = 'good'
imageurl = "https://i.imgur.com/yLPKBlF.png"
elif state == 'UP':
color = 'good'
imageurl = "https://i.imgur.com/yLPKBlF.png"
elif state == 'WARNING':
color = 'warning'
imageurl = "https://i.imgur.com/GIv0R4o.png"
elif state == 'DOWN':
color = 'attention'
imageurl = "https://i.imgur.com/C2B8moA.png"
elif state == 'CRITICAL':
color = 'attention'
imageurl = "https://i.imgur.com/C2B8moA.png"
elif state == 'UNKNOWN':
color = 'light'
imageurl = "https://i.imgur.com/ALr2Cwx.png"
elif state == 'UNREACHABLE':
color = 'light'
imageurl = "https://i.imgur.com/ALr2Cwx.png"
else:
color = 'dark'
imageurl = "https://i.imgur.com/ALr2Cwx.png"
# Check if type is custom
if comment or author:
iscomment = "true"
else:
iscomment = "false"
headers = {
'Content-Type': 'application/json;charset=utf-8',
}
json_data = {
"type":"message",
"attachments":[
{
"contentType":"application/vnd.microsoft.card.adaptive",
"contentUrl":"null",
"content":{
"$schema":"http://adaptivecards.io/schemas/adaptive-card.json",
"type":"AdaptiveCard",
"version":"1.3",
"title":"Monitoring",
"msteams": {
"width": "Full"
},
"body":[
{
"type": "Container",
"items": [
{
"type": "TextBlock",
"text": notificationtype,
"weight": "bolder",
"size": "medium",
"isSubtle": "true",
"spacing": "None"
},
{
"type": "ColumnSet",
"columns": [
{
"type": "Column",
"width": "auto",
"items": [
{
"type": "Image",
"url": imageurl,
"altText": state,
"size": "small",
"style": "person"
}
]
},
{
"type": "Column",
"width": "stretch",
"items": [
{
"type": "RichTextBlock",
"inlines": [
{
"type": "TextRun",
"text": hostdisplayname,
"weight": "bolder",
"size": "large",
"wrap": "true"
},
{
"type": "TextRun",
"text": " is ",
"weight": "bolder",
"wrap": "true",
"isSubtle": "true"
},
{
"type": "TextRun",
"text": state,
"weight": "bolder",
"size": "large",
"wrap": "true"
}
]
},
{
"type": "TextBlock",
"spacing": "none",
"text": current_datetime_str,
"isSubtle": "true",
"wrap": "true"
}
]
}
]
}
]
},
{
"type": "Container",
"items": [
{
"type": "RichTextBlock",
"inlines": [
{
"type": "TextRun",
"text": output
}
]
},
{
"type": "FactSet",
"isVisible": iscomment,
"facts": [
{
"title": "Comment:",
"value": comment
},
{
"title": "Author:",
"value": author
}
]
}
]
}
],
"actions": [
{
"type": "Action.OpenUrl",
"url": icingaweburl+"/icingadb/host?name="+hostname+"",
"title": "View"
}
]
}
}
]
}
response = requests.post(
boturl,
headers=headers,
json=json_data,
verify=False
)